▶현재 시간 : 2022년 10월 25일 18:13 p.m.
omg. 퀴즈 너무 어렵네.
내 생각엔.. 최소 3번 이상 따라해 보면서 해야지 이해 할듯..
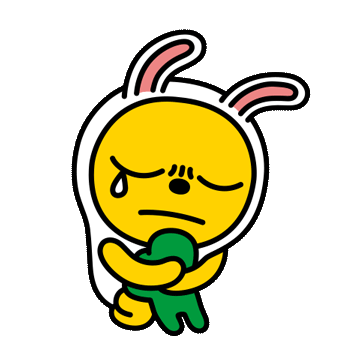
▶퀴즈: 객체지향요구사항
- 사람은 자식, 부모님, 조부모님이 있다.
- 모든 사람은 이름, 나이, 현재 장소정보(x,y좌표)가 있다.
- 모든 사람은 걸을 수 있다. 장소(x, y좌표)로 이동한다.
- 자식과 부모님은 뛸 수 있다. 장소(x, y좌표)로 이동한다.
- 조부모님의 기본속도는 1이다. 부모의 기본속도는 3, 자식의 기본속도는 5이다.
- 뛸때는 속도가 기본속도대비 +2 빠르다.
- 수영할때는 속도가 기본속도대비 +1 빠르다.
- 자식만 수영을 할 수 있다. 장소(x, y좌표)로 이동한다.
위 요구사항을 만족하는 클래스들을 바탕으로, Main 함수를 다음 동작을 출력(System.out.println)하며 실행하도록 작성하시오. 이동하는 동작은 각자 순서가 맞아야 합니다.
- 모든 종류의 사람의 인스턴스는 1개씩 생성한다.
- 모든 사람의 처음 위치는 x,y 좌표가 (0,0)이다.
- 모든 사람의 이름, 나이, 속도, 현재위치를 확인한다.
- 걸을 수 있는 모든 사람이 (1, 1) 위치로 걷는다.
- 뛸 수 있는 모든 사람은 (2,2) 위치로 뛰어간다.
- 수영할 수 있는 모든 사람은 (3, -1)위치로 수영해서 간다.
객체지향에서 배운 개념과 문법을 이용해서 다음 요구조건을 만족하는 클래스를 작성하시요. 여러분이 게임을 만든다고 생각해보세요.
▶정답
- Human java.
public class Human {
String name;
int age;
int speed;
int x,y;
public Human(String name, int age, int speed, int x, int y) {
this.name = name;
this.age = age;
this.speed = speed;
this.x = x;
this.y = y;
}
public Human(String name, int age, int speed) {
this(name, age, speed, 0,0) ;
}
public String getLocation() {
return "( "+ x + ", " + y + ")";
}
protected void printWhoAmI(){
System.out.println("My name is " + name + ". " + age + " aged.");
}
}
Walkable.java
public interface Walkable {
void walk(int x, int y);
}
Runnable.java
public interface Runnable {
void run(int x, int y);
}
Swimmable.java
public interface Swimmable {
void swim(int x, int y);
}
GrandParents.java
public class GrandParent extends Human implements Walkable{
public GrandParent(String name, int age) {
super(name, age, 1 );
}
@Override
public void walk(int x, int y) {
printWhoAmI();
System.out.println("walk speed: " + speed);
this.x = x;
this.y = y;
System.out.println("walked to" + getLocation());
}
}
Parents.java
public class Parent extends Human implements Walkable, Runnable{
public Parent(String name, int age) {
super(name, age, 3);
}
@Override
public void run(int x, int y) {
printWhoAmI();
System.out.println("run speed: " + speed+ 2);
this.x = x;
this.y = y;
System.out.println("ran to" + getLocation());
}
@Override
public void walk(int x, int y) {
printWhoAmI();
System.out.println("walk speed: " + speed);
this.x = x;
this.y = y;
System.out.println("walked to" + getLocation());
}
}
Child.java
public class Child extends Human implements Walkable, Runnable, Swimmable{
public Child(String name, int age) {
super(name, age, 5 );
}
@Override
public void swim(int x, int y) {
printWhoAmI();
System.out.println("swim speed: " + speed+ 1);
this.x = x;
this.y = y;
System.out.println("swum to" + getLocation());
}
@Override
public void run(int x, int y) {
printWhoAmI();
System.out.println("run speed: " + speed+ 2);
this.x = x;
this.y = y;
System.out.println("ran to" + getLocation());
}
@Override
public void walk(int x, int y) {
printWhoAmI();
System.out.println("walk speed: " + speed);
this.x = x;
this.y = y;
System.out.println("walked to" + getLocation());
}
}
Main.java
public class Main {
public static void main(String[] args) {
Human grandParent = new GrandParent( "할아버지", 70);
Human parent = new Parent("엄마", 50);
Human child = new Child( "나", 20);
Human[] humans = {grandParent, parent, child};
for(Human human: humans){
System.out.println(human.name + ",나이: " +human.age + ",속도: "+ human.speed + ", 현재위치" + human.getLocation());
}
System.out.println("<활동 시작>");
for(Human human : humans){
if(human instanceof Walkable) {
((Walkable) human).walk(1, 1);
System.out.println("------");
}
if(human instanceof Runnable){
((Runnable) human).run(2,2);
System.out.println("------");
}
if(human instanceof Swimmable){
((Swimmable) human).swim(3,-1);
System.out.println("------");
}
}
}
}
'Java' 카테고리의 다른 글
Java - 변수 기본 타입 (0) | 2022.11.08 |
---|---|
Java - 변수 (0) | 2022.11.08 |
Java - 객체지향언어 (추상클래스) (0) | 2022.10.25 |
Java - 객체지향언어 (접근 제어자) (0) | 2022.10.25 |
Java - 오버로딩 vs 오버라이딩 (0) | 2022.10.25 |